composer require shopwwi/webman-search
xunsearch 使用讯搜搜索选定器
composer require hightman/xunsearch
meilisearch 使用meilisearch选定器
composer require meilisearch/meilisearch-php
elasticsearch 使用elasticsearch选定器
composer require elasticsearch/elasticsearch
meilisearch 安装方法查看 https://docs.meilisearch.com/learn/getting_started/quick_start.html
xunsearch 安装方法查看 http://www.xunsearch.com/doc/php/guide/start.installation
elasticsearch 安装方法查看 https://www.elastic.co/
php webman shopwwi:search
use \Shopwwi\WebmanSearch\Facade\Search;
// id为主键字段 index为索引
$search = Search::use('meilisearch',['id'=>'id','index'=>'index']);
php webman search:create:index // es搜索必须先执行此命令创建索引
php webman search:update:index // 更新索引配置
php webman search:drop:index //删除索引
- 写入文档
```php
$data = [
['id' => 1, 'title' => '我从来都不曾承认我其实是个大帅哥' ,'create_at' => 2022-03-24 08:08:08,'type' => 'A'],
['id' => 2, 'title' => '时间万物除我之外' ,'create_at' => 2022-03-24 09:08:08,'type' => 'B'],
['id' => 3, 'title' => '你看见我的小熊了吗?' ,'create_at' => 2022-03-24 10:08:08,'type' => 'B'],
['id' => 4, 'title' => '这是一个神奇的世界,因为你永远不会知道我在哪' ,'create_at' => 2022-03-24 10:08:08,'type' => 'C']
]
$search->create($data); //批量写入 支持单条 写入一维数组即可
$data = [
['id' => 3, 'title' => '你看见我的小熊了吗?哈哈哈']
];
$search->update($data); // 批量修改 主键必须存在 单条修改写入一维数组
$search->destroy(3); //则会删除id为3的数据
$search->destroy([2,3]); //批量删除 id为2和3的数据
$search->clear();
use \Shopwwi\WebmanSearch\Facade\Search;
// 基础查询关键词
$result = $search->q('我')->get();
// 全部查询 默认只显示20条
$result = $search->get();
//指定字段(默认指定所有)
$result = $search->select(['id','title'])->get();
//限定查询数量 默认为20
$result = $search->limit(100)->q('我')->get();
//带条件搜索(一定要设定可检索字段 不然无效,一般在初始化新增之后)
// where($column, $operator = null, $value = null, string $boolean = 'AND') static 搜索
// orWhere($column, $operator = null, $value = null) static 搜索或者
Search::updateFilterableAttributes(['id','title','type','create_at']);
$result = $search->where('type','B')->limit(100)->q('我')->get();
$result = $search->where('type','!=','B')->limit(100)->q('我')->get();
$result = $search->orWhere('type','B')->limit(100)->q('我')->get();
// whereIn($column, $values, string $boolean = 'AND', bool $not = false) static 存在当中
// orWhereIn(string $column, $values) static 搜索或者存在当然
// whereNotIn(string $column,array $values, string $boolean = 'AND') static 搜索不存在当中
// orWhereNotIn(string $column, $values) static 搜索或者不存在当中
$result = $search->whereIn('type',['A','B'])->limit(100)->q('我')->get();
$result = $search->whereNotIn('type',['A','B'])->limit(100)->q('我')->get();
$result = $search->orWhereIn('type',['A','B'])->limit(100)->q('我')->get();
$result = $search->orWhereNotIn('type',['A','B'])->limit(100)->q('我')->get();
// whereRaw($sql, $boolean = 'AND') static 原生数据查询
$result = $search->where('type','B')->whereRaw('(id = 1 OR id = 2)')->limit(100)->q('我')->get();
//whereBetween($column,array $values, string $boolean = 'AND')
$result = $search->whereBetween('id',[1,5])->limit(100)->q('我')->get();
//如果您的文档包含_geo数据,您可以使用_geoRadius内置过滤规则根据其地理位置过滤结果
$result = $search->where('type','B')->whereRaw('_geoRadius(45.4628328, 9.1076931, 2000)')->limit(100)->q('我')->get();
// 分页
$result = $search->where('type','B')->limit(20)->q('我')->paginate(\request()->input('page',1));
//关键词高亮
$result = $search->where('type','B')->limit(20)->highlight(['title'])->q('我')->paginate(\request()->input('page',1));
获取建议词(如搜索s则会出现与s相关的汉字数组 ,meilisearch不支持,es请使用原sdk方法查询)
$result = $search->limit(20)->q('s')->suggest();// 不带统计数,数量都为0
$result = $search->limit(20)->q('s')->suggest(true); // 带统计数
$result = $search->first(2);
// 字段排序(一定要设定可排序字段 不然无效,一般在初始化新增之后)
//1.使用orderBy方法
$result = $search->where('type','B')->orderBy('create_at','desc')->orderBy('id')->limit(100)->q('我')->get();
//2.全局设定
$result = $search->where('type','B')->limit(100)->q('我')->get();
获取原SDK方法(各方法请查看各选定器包说明文档)
//返回所选定器本身SDK方法
$search = Search::use('meilisearch',['id'=>'id','index'=>'index'])->us();
//如meilisearch调用任务方法
$search->getTasks(); // 查询所有任务
收藏,后面估计要用到了
不错
正好可以试一下,这个比es小巧一些。
我的规模也不大。
不错。
收藏,点赞加转发。
收藏备用
收藏备用
很棒👍
一个字:牛
👍
收藏
直接运行没问题 打包Phar后出现找不到类的情况
Class 'Shopwwi\WebmanSearch\Command\SearchCreateIndexCommand' not found in phar:
看看是否打包时排除了command目录
看配置文件应该是没有被排除的 现在只有把command里的四个命令都取消了phar包才能正常运行
并且看了phar包里 composer相关依赖都是有的
这个是phar包下vendor
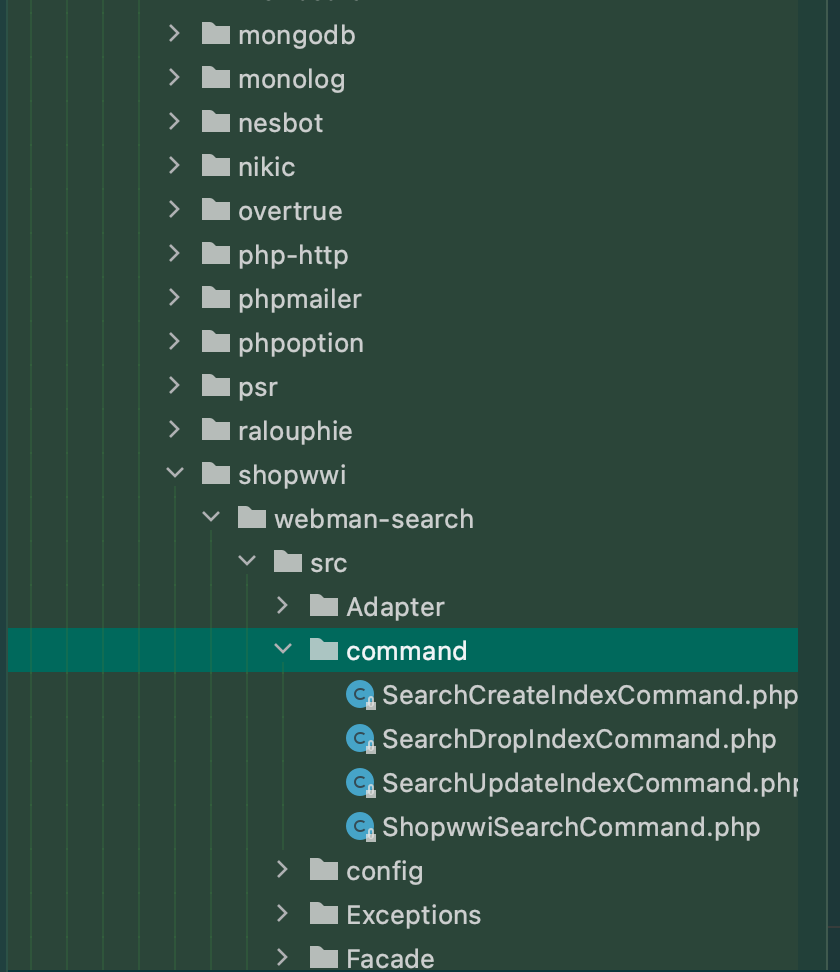
喔 我的错 大小写引起的问题 马上修复 等会更新版本即可
ok
请更新至v1.0.4试试 如果有问题及时反馈 谢谢
更新到v1.0.4之后正常了 感谢
请教下 这个应该如何解决呢
这个没看出来是啥问题 是不是数据格式不正确?
数据就是普通一维数组 现在就是普通测试一下 我感觉可能是 es 版本问题 还在定位问题
切换到7.17.4 es版本就正常了 可能新版本对调用方式做了一些新的校验
这个request()会导致在websocket模式下调用拿不到request对象 要注入一下request对象吧
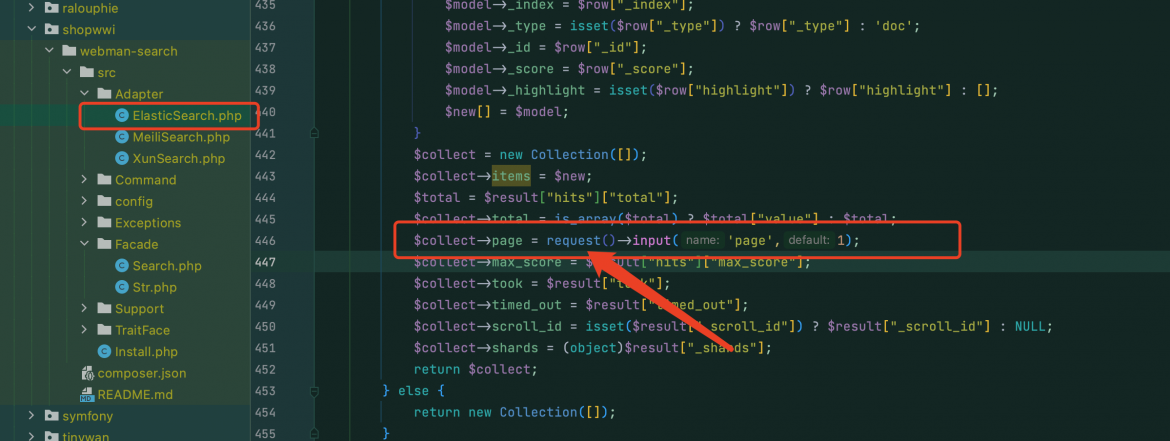
$collect->page = request() ? request()->input('page',1) : 1;
给加个三元吧 球球了
这样加上也解决不了分页切换的问题呀
所以要不改写下page可传参,我这里改好之后提个PR?
可以的
PR已申请
已合并 并更新
Fatal error: Declaration of Elastic\Elasticsearch\Response\Elasticsearch::withStatus(int $code, string $reasonPhrase = ''): Psr\Http\Message\Resp
onseInterface must be compatible with Psr\Http\Message\ResponseInterface::withStatus($code, $reasonPhrase = '') in D:\php_project\dianwai_api\wz_
im_message\vendor\elasticsearch\elasticsearch\src\Response\Elasticsearch.php on line 87
Worker process terminated with ERROR: E_COMPILE_ERROR "Declaration of Elastic\Elasticsearch\Response\Elasticsearch::withStatus(int $code, string
$reasonPhrase = ''): Psr\Http\Message\ResponseInterface must be compatible with Psr\Http\Message\ResponseInterface::withStatus($code, $reasonPhra
se = '') in D:\php_project\dianwai_api\wz_im_message\vendor\elasticsearch\elasticsearch\src\Response\Elasticsearch.php on line 87"
process D:\php_project\dianwai_api\wz_im_message\start.php terminated and try to restart
https://github.com/elastic/elasticsearch-php/issues/1318
看看相同问题 尝试解决
换了7.17.4就好了
小项目想直接用xunsearch,报错。
PHP 8.1
webman 1.5.6
hightman/xunsearch 1.4.7
xunsearch-server 1.4.17
在config/.../search/下新建ini/goods.ini
业务代码
PHP Fatal error: During inheritance of ArrayAccess: Uncaught [XSErrorException] vendor/hightman/xunsearch/lib/XSDocument.class.php(300): Return type of XSDocument::offsetGet($name) should either be compatible with ArrayAccess::offsetGet(mixed $offset): mixed, or the #[\ReturnTypeWillChange] attribute should be used to temporarily suppress the notice(8192) in /www/wwwroot/admin.webman.com/vendor/hightman/xunsearch/lib/XSDocument.class.php on line 43
Fatal error: During inheritance of ArrayAccess: Uncaught [XSErrorException] vendor/hightman/xunsearch/lib/XSDocument.class.php(300): Return type of XSDocument::offsetGet($name) should either be compatible with ArrayAccess::offsetGet(mixed $offset): mixed, or the #[\ReturnTypeWillChange] attribute should be used to temporarily suppress the notice(8192) in /www/wwwroot/admin.webman.com/vendor/hightman/xunsearch/lib/XSDocument.class.php on line 43
Worker[1235] process terminated with ERROR: E_ERROR "During inheritance of ArrayAccess: Uncaught [XSErrorException] vendor/hightman/xunsearch/lib/XSDocument.class.php(300): Return type of XSDocument::offsetGet($name) should either be compatible with ArrayAccess::offsetGet(mixed $offset): mixed, or the #[\ReturnTypeWillChange] attribute should be used to temporarily suppress the notice(8192) in /www/wwwroot/admin.webman.com/vendor/hightman/xunsearch/lib/XSDocument.class.php on line 43"
worker[webman:1235] exit with status 65280
这个elasticsearch 有账号密码 在配置文件需要怎么传入